將 Facebook、Twitter 和 RSS 社交統計與 jQuery、PHP 和 YQL 相結合
隨著我們越來越依賴越來越多的社交服務,越來越需要提供一種簡單的方式讓我們的網站訪問者參與到我們多樣化的社交活動中。
在本教程中,我們將創建一個簡單的小部件,它結合了您的 RSS 閱讀器、推特關注者和您的 facebook 粉絲頁面的粉絲數量,以粗略估計您的社交受歡迎程度。
我們使用 jQuery 和 tipTip 插件、面向對象的 PHP 和 Yahoo 的 YQL,同時展示了一些有趣的 Web 開發技術。
第 1 步 - PHP
YQL 是一個免費的 Yahoo Web 服務,它使我們能夠通過一致的類似 SQL 的語言(因此得名)與眾多第三方 API 進行通信。它基本上是位於您和其他 API 之間的網關。
這在這裡尤其重要,因為我們使用 Yahoo 的 YQL 來完成三個非常不同的任務:
- 使用 FeedBurner 的感知 API(以必須解析的 XML 文件的形式提供)獲取您的 RSS 訂閱者數量。注意: 您必須啟用感知 API 才能將此小部件與您自己的提要一起使用。這是在您的供稿設置頁面上的“宣傳”選項卡中完成的;
- 使用 twitter 的 API 獲取您的關注者數量;
- 使用 Facebook 的新 Graph API (鏈接)以獲取有關您的 Facebook 粉絲頁面的粉絲數量的信息。
如果不是 YQL,我們必須研究和實施三種截然不同的解決方案,這會大大減慢我們的速度。
包括/subscriber_stats.class.php
class SubscriberStats{ public $twitter,$rss,$facebook; public $services = array(); public function __construct($arr){ $this->services = $arr; $yqlQueries = array(); // Forming the Feedburner Awaraness API URL from the passed feed URL: $feedBurnerAwarenessAPI = 'http://feedburner.google.com/api/awareness'. '/1.0/GetFeedData?uri='.end(split('/',trim($arr['feedBurnerURL'],'/'))); // Building an array with queries: if($arr['feedBurnerURL']) $yqlQueries[] = ' SELECT * FROM xml WHERE url=\''.$feedBurnerAwarenessAPI.'\' '; if($arr['twitterName']) $yqlQueries[] = ' SELECT * FROM twitter.user.profile WHERE id=\''.$arr['twitterName'].'\' '; if($arr['facebookFanPageURL']) $yqlQueries[] = ' SELECT likes FROM facebook.graph WHERE id=\''.end(split('/',trim($arr['facebookFanPageURL'],'/'))).'\' '; // Combing them into a YQL multiquery: $multiQuery = 'SELECT * FROM query.multi WHERE queries = "'.join(';',$yqlQueries).'"'; // Executing the query: $result = json_decode( file_get_contents('http://query.yahooapis.com/v1/public/yql?q='. urlencode($multiQuery).'&format=json&diagnostics=false&' 'amp;env=store%3A%2F%2Fdatatables.org%2Falltableswithkeys') )->query->results->results; // The results from the queries are accessible in the $results array: $this->rss = $result[0]->rsp->feed->entry->circulation; $this->twitter = $result[1]->item->meta[5]->content; $this->facebook = $result[2]->json->fan_count; } public function generate(){ $total = number_format($this->rss+$this->twitter+$this->facebook); echo ' <div class="subscriberStats"> <div class="subscriberCount" title="'.$total.'+ Total Social Media Followers">'.$total.'</div> <div class="socialIcon" title="'.number_format($this->rss).' RSS Subscribers"> <a href="'.$this->services['feedBurnerURL'].'"> <img src="img/rss.png" alt="RSS" /></a> </div> <div class="socialIcon" title="'.number_format($this->facebook).' Fans on Facebook"> <a href="'.$this->services['facebookFanPageURL'].'"> <img src="img/facebook.png" alt="Facebook" /></a> </div> <div class="socialIcon" title="'.number_format($this->twitter).' Twitter Followers"> <a href="http://twitter.com/'.$this->services['twitterName'].'"> <img src="img/twitter.png" alt="Twitter" /></a> </div> </div> '; } }
當我們創建這個類的對象時,調用construct方法,創建並執行一個YQL查詢。
要從 YQL 的服務器請求數據,我們只需使用 file_get_contents() 將查詢作為 URL 地址的參數傳遞。這將返回一個 JSON 對象(基本上是一個 JavaScript 對象),我們可以使用內置 json_decode() 將其解碼為原生 PHP 數組 功能。
這些查詢的結果保存在本地,並可供 generate() 使用 呈現所有需要的標記的方法。
現在讓我們看看這個類是如何使用的:
subscriber_count.php
require "includes/subscriber_stats.class.php"; $cacheFileName = "cache.txt"; // IMPORTANT: after making changes to this file (or the SubscriberStats class) // remeber to delete cache.txt from your server, otherwise you wont see your changes. // If a cache file exists and it is less than 6*60*60 seconds (6 hours) old, use it: if(file_exists($cacheFileName) && time() - filemtime($cacheFileName) > 6*60*60) { $stats = unserialize(file_get_contents($cacheFileName)); } if(!$stats) { // If no cache was found, fetch the subscriber stats and create a new cache: $stats = new SubscriberStats(array( 'facebookFanPageURL' => 'http://www.facebook.com/smashmag', 'feedBurnerURL' => 'http://feeds.feedburner.com/Tutorialzine', 'twitterName' => 'Tutorialzine' )); // Serialize turns the object into a string, // which can later be restored with unserialize(): file_put_contents($cacheFileName,serialize($stats)); } // You can access the individual stats like this: // $stats->twitter; // $stats->facebook; // $stats->rss; // Output the markup for the stats: $stats->generate();
向 YQL 的服務器發送查詢並接收響應是一個相對緩慢的過程,並且在每次頁面加載時都請求相同的信息是不明智的(更不用說我們可能會因濫用 API 而被禁止)。
這就是我們實現簡單緩存系統的原因。這個想法很簡單:如果緩存文件不存在(或超過 6 小時),連接到 YQL,創建一個新的緩存文件並輸出 XHTML 標記。否則,直接讀取緩存並輸出即可。這樣,我們每六個小時只向 API 發送一次請求,非常適合任何實際用途。
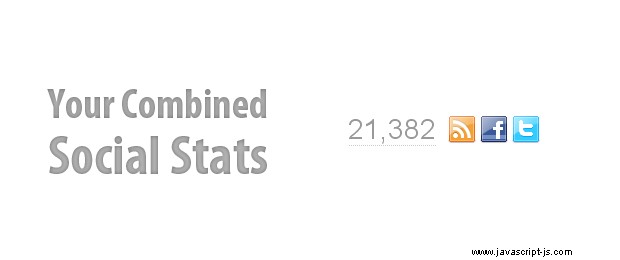
第 2 步 - XHTML
如上面 PHP 部分所述,generate() 方法呈現用於顯示統計信息的所有 XHTML 標記。生成的代碼如下所示:
示例代碼
<div class="subscriberStats"> <div class="subscriberCount" title="25,382+ Total Social Media Followers>25,382</div> <div class="socialIcon" title="5,921 RSS Subscribers"> <a href="http://feeds.feedburner.com/Tutorialzine"> <img alt="RSS" src="img/rss.png" /></a> </div> <div class="socialIcon" title="16,813 Fans on Facebook"> <a href="http://www.facebook.com/smashmag"> <img alt="Facebook" src="img/facebook.png" /></a> </div> <div class="socialIcon" title="2,648 Twitter Followers"> <a href="http://twitter.com/Tutorialzine"> <img alt="Twitter" src="img/twitter.png" /></a> </div> </div>
此代碼通過 AJAX 獲取並顯示在頁面上。注意標題屬性。它們被用作jQuery和tipTip插件創建的精美工具提示的內容,我們稍後會討論。
第 3 步 - CSS
CSS 代碼也非常簡單明了。 subscriberStats 是主要的外部 div,在裡面我們有許多 .socialIcon div 和 subscrberCount .
css/styles.css
.subscriberStats{ height:35px; padding:5px; width:220px; } .socialIcon{ float:left; height:32px; width:32px; } a img{ border:none; } .subscriberCount{ border-bottom:1px dotted #CCCCCC; color:#999999; float:left; font-size:28px; line-height:32px; margin-right:10px; } #main{ height:100px; margin:140px auto 50px; position:relative; width:200px; }
所有這些都向左浮動。另請注意,我們在第 14 行禁用了圖標圖像的邊框,這些邊框默認顯示並破壞了您的設計。
第 4 步 - jQuery
將 jQuery 庫包含到頁面後,我們只需要監聽 $(document).ready 事件,該事件在頁面的所有標記都可以訪問時執行(這發生在加載圖像等內容之前,並且比onload 事件)。
js/script.js
$(document).ready(function(){ // Using the load AJAX method to fetch the subscriber markup // from subscriber_count.php: $('#main').load('subscriber_count.php',function(){ // Once loaded, convert the title attributes to tooltips // with the tipTip jQuery plugin: $('.subscriberStats div').tipTip({defaultPosition:'top'}); }) });
#main 是我們要插入統計信息的 div。這可能是您的側邊欄或網站標題。 load 方法從 suscriber_count.php 獲取標記 並將其顯示在頁面上。在此之後調用回調函數,所有 div 標題都被 tipTip 插件替換為精美的工具提示 .
這樣我們的綜合社交統計小部件就完成了!
結論
像 YQL 這樣的服務與第三方 API 一起工作是一種魅力。它不僅為大量技術提供了一個通用接口,而且還保證即使底層 API 隨時間發生變化,您也可以訪問所需的服務。
你怎麼看?您將如何改進此代碼?