快速提示:使用 JavaScript 檢測您的位置
大多數現代設備都能夠通過 GPS、WiFi 或 IP 地理定位來檢測自己的位置。開發者可以使用這些信息來提供更好的搜索建議、附近的商店位置,並在他們的應用和網站中實現各種有用的地圖交互。
在下面的文章中,我們將介紹一種簡單的純 JavaScript 方式來訪問設備的行踪,而無需依賴任何外部依賴項或第三方服務。開始吧!
位置來源
JavaScript 以 Geolocation API 的形式提供了一個簡單但功能強大的設備定位工具。它由一小組易於使用的方法組成,可以通過前面提到的所有三個服務獲取設備位置:
- GPS - 主要在移動設備上,精確到 10 米。
- WiFi - 在大多數連接互聯網的設備上都可用,而且非常準確。
- IP 地理定位 - 僅限於區域,通常不可靠,用作其他兩個失敗時的最壞情況。
當請求地理數據時,瀏覽器將根據可用的內容嘗試並使用上述所有三個選項。通常使用來自 WiFi 源的結果,因為它比 GPS 更快,並且比 IP 地理定位更準確。
使用地理定位 API
Geolocation API 具有幾乎完整的跨瀏覽器支持,但為了確保我們的用戶可以訪問它,最好在執行任何操作之前檢查 geolocation
Window.navigator
中存在對象 界面。
if (navigator.geolocation) { // geolocation is available } else { // geolocation is not supported }
navigator.geolocation
內部 對象駐留 API 的所有方法:
Geolocation.getCurrentPosition()
- 確定設備的當前位置。Geolocation.watchPosition()
- 監聽位置變化並在每次移動時調用回調。Geolocation.clearWatch()
- 刪除watchPosition
事件處理程序。
Тhe getCurrentPosition()
和 watchPosition()
方法以幾乎相同的方式使用。它們都是異步工作的,嘗試獲取設備位置,並根據嘗試的結果調用成功回調或錯誤回調(如果提供)。
navigator.geolocation.getCurrentPosition( // Success callback function(position) { /* position is an object containing various information about the acquired device location: position = { coords: { latitude - Geographical latitude in decimal degrees. longitude - Geographical longitude in decimal degrees. altitude - Height in meters relative to sea level. accuracy - Possible error margin for the coordinates in meters. altitudeAccuracy - Possible error margin for the altitude in meters. heading - The direction of the device in degrees relative to north. speed - The velocity of the device in meters per second. } timestamp - The time at which the location was retrieved. } */ }, // Optional error callback function(error){ /* In the error object is stored the reason for the failed attempt: error = { code - Error code representing the type of error 1 - PERMISSION_DENIED 2 - POSITION_UNAVAILABLE 3 - TIMEOUT message - Details about the error in human-readable format. } */ } );
如您所見,使用 Geolocation API 非常簡單。我們只需要調用正確的方法,等待它返回坐標,然後對它們做任何我們想做的事情。
用戶權限
由於 Geolocation API 暴露了很深的個人信息,當應用程序第一次嘗試訪問它時,會彈出一個對話框請求權限。這樣可以確保用戶不會洩露他們的私人數據,除非他們明確允許。
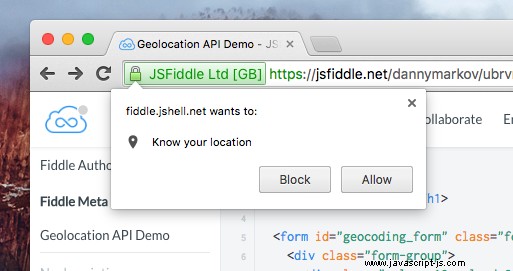
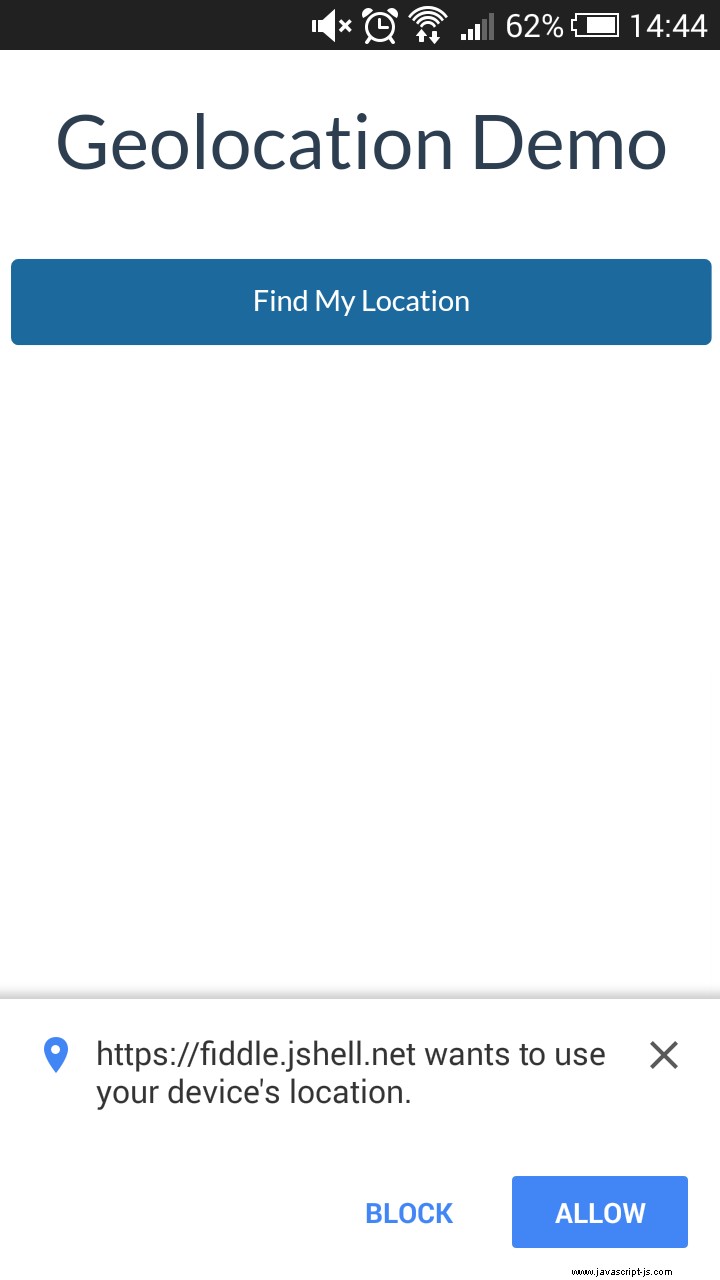
瀏覽器通常負責顯示對話框,但開發人員也可以通過編程方式請求權限。這有時是必要的,因為一旦被拒絕,原始瀏覽器生成的對話框就不會再次顯示。
安全主機
另一項保護措施是使用 HTTPS 連接。由於新的網絡安全政策,Google Chrome(桌面版和移動版)不再允許非安全主機運行 Geolocation API。相反,想要使用此功能的開發人員必須通過 HTTPS 為他們的應用程序提供服務,從而最大限度地降低人們數據被盜或濫用的風險。
您可以在此 Google Developers 博客文章中閱讀有關此問題的更多信息。
演示應用
為了演示整個過程是如何工作的,我們構建了一個過於簡化的應用程序來展示 API 的一些功能。它由一個按鈕組成,當按下該按鈕時,它會抓取設備坐標並將它們提供給這個 GMaps 插件,從而在地圖上精確定位。
findMeButton.on('click', function(){ navigator.geolocation.getCurrentPosition(function(position) { // Get the coordinates of the current position. var lat = position.coords.latitude; var lng = position.coords.longitude; // Create a new map and place a marker at the device location. var map = new GMaps({ el: '#map', lat: lat, lng: lng }); map.addMarker({ lat: lat, lng: lng }); }); });
JSFiddle 上提供了演示以及完整代碼:
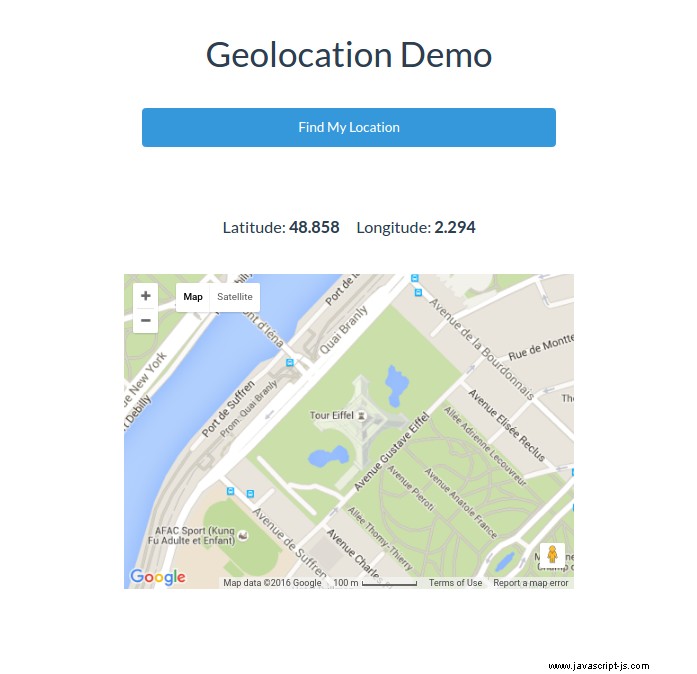
結論
Gelocation API 可靠且可用於生產,因此我們鼓勵您試用它並了解它的功能。如果您在網絡上看到了該技術的一些原始用途,或者您自己製作了一個很酷的項目,請在下面的評論部分隨意分享 - 我們很樂意看到它!